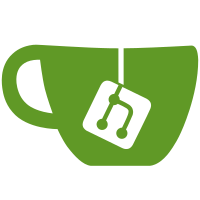
2 changed files with 97 additions and 0 deletions
@ -0,0 +1,36 @@
|
||||
package Text::Dokuwiki::Render; |
||||
|
||||
use strict; |
||||
use warnings; |
||||
use utf8; |
||||
|
||||
sub new { |
||||
my ($class) = @_; |
||||
my $self = {}; |
||||
|
||||
return bless($self, $class); |
||||
} |
||||
|
||||
sub treewalk { |
||||
my ($self, @tree) = @_; |
||||
my $output = ""; |
||||
|
||||
foreach my $part (@tree) { |
||||
if (ref $part eq 'ARRAY') { |
||||
my ($tag, $attrs, @rest) = @{$part}; |
||||
if ($self->SUPER::can($tag)) { |
||||
$output .= $self->$tag($attrs, $self->treewalk(@rest)); |
||||
} elsif ($self->SUPER::can('default')){ |
||||
$output .= $self->default($tag, $attrs, $self->treewalk(@rest)); |
||||
} else { |
||||
die("Unimplemented handler for tag: $tag\n"); |
||||
} |
||||
next; |
||||
} |
||||
$output .= $part; |
||||
} |
||||
|
||||
return $output; |
||||
} |
||||
|
||||
1; |
@ -0,0 +1,61 @@
|
||||
package Text::Dokuwiki::Render::HTML; |
||||
|
||||
use strict; |
||||
use warnings; |
||||
use utf8; |
||||
|
||||
use base 'Text::Dokuwiki::Render'; |
||||
|
||||
my $noclose = { |
||||
br => 1, |
||||
hr => 1, |
||||
}; |
||||
|
||||
my $display = { |
||||
div => 'block', |
||||
ul => 'block', |
||||
h1 => 'line', |
||||
h2 => 'line', |
||||
h3 => 'line', |
||||
p => 'line', |
||||
br => 'block', |
||||
}; |
||||
|
||||
sub _escape { |
||||
my ($text) = @_; |
||||
|
||||
$text =~ s{\\}{\\\\}go; |
||||
$text =~ s{"} {\\"}go; |
||||
return $text; |
||||
} |
||||
|
||||
sub _attrs { |
||||
my ($attrs) = @_; |
||||
my $text = ""; |
||||
|
||||
while (my ($key, $value) = each($attrs)) { |
||||
$text .= sprintf ' %s="%s"', $key, _escape($value); |
||||
} |
||||
|
||||
return $text; |
||||
} |
||||
|
||||
sub default { |
||||
my ($self, $tag, $attrs, $content) = @_; |
||||
my $c = $noclose->{$tag} // 0; |
||||
my $d = $display->{$tag} // 'inline'; |
||||
|
||||
my $text = "<$tag" . _attrs($attrs) . ">"; |
||||
$text .= "\n" if ($d eq 'block'); |
||||
return $text if ($c); |
||||
|
||||
$text .= $content; |
||||
$text .= "\n" if ($d eq 'block'); |
||||
|
||||
$text .= "</$tag>"; |
||||
$text .= "\n" if ($d eq 'block' or $d eq 'line'); |
||||
|
||||
return $text; |
||||
} |
||||
|
||||
1; |
Loading…
Reference in new issue